这款激光是一种同轴激光,白光源,相对于三角反射理激光有其自身的优点。
如果是双头来测厚的话,有几点关键的问题:
激光点同轴度必须保证对齐
双激光头如何保证数据的同步性
激光的有效量程必须大于被测物运动时的波浪
代码如下。
有几点说明:
(1)程序用到了netMarketing类库
(2)由于使用了tcp方式访问激光头,程序上要保证两个激光头是同步发起读动作
using netMarketing.http; using netMarketing.Net.NetSocket; using netMarketing.thread; using sharClass; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading; using System.Threading.Tasks; using System.Windows.Forms; namespace zw7000test { public partial class Form1 : Form { private winData configdata = new winData(); public Form1() { InitializeComponent(); Control.CheckForIllegalCrossThreadCalls = false; } private void Form1_Load(object sender, EventArgs e) { if (workInfoFromDisk()) { tbSum.Text = configdata.dataSum.ToString(); tbLaserIP.Text = configdata.laserIP; tbLaserPort.Text = configdata.laserPort.ToString(); } Thread th_readValue = new Thread(Read); th_readValue.IsBackground = true; th_readValue.Start(); } private void Read() { while (true) { //上激光 string lasername = ""; lasername = "上激光"; laserBufferUp.Clear(); if (!isConnectUp) { try { InitPort("192.168.250.51", configdata.laserPort, Laser.Up); outmsg(lasername + "初始化成功!\r\n"); var bary = binHelper.toBin(string.Format("LS 1 {0}\r", 10)); var buffer = new byte[] { }; SendAndGetData(bary, out buffer, (uint)bary.Length, Laser.Up); outmsg("读取数据 " + new string(Encoding.Default.GetChars(buffer))); } catch (Exception ex) { outmsg(ex.Message + Environment.NewLine); return; } } // ShowAlarm.Delay(100); //等待激光读取完成 var bary1 = binHelper.toBin(string.Format("MS 0 0\r")); byte[] buffer1 = new byte[] { }; SendAndGetData(bary1, out buffer1, (uint)1 * 12, Laser.Up); var str1 = new string(Encoding.Default.GetChars(buffer1)); double value = 0; if (str1.Contains("-----------")) //超出范围 { tbx_upLaser.Text = "NA"; } else { var listtmp1 = str1.Split(new string[] { "," }, StringSplitOptions.RemoveEmptyEntries).ToList().ConvertAll((s) => double.Parse(s)); //格式化成mm value = Convert.ToInt32(listtmp1[0]); value = value / 1000000; tbx_upLaser.Text = value.ToString(); } //下激光 lasername = ""; lasername = "下激光"; laserBufferUp.Clear(); if (!isConnectDown) { try { InitPort("192.168.250.50", configdata.laserPort, Laser.Down); outmsg(lasername + "初始化成功!\r\n"); var bary = binHelper.toBin(string.Format("LS 1 {0}\r", 10)); var buffer = new byte[] { }; SendAndGetData(bary, out buffer, (uint)bary.Length, Laser.Down); outmsg("读取数据 " + new string(Encoding.Default.GetChars(buffer))); } catch (Exception ex) { outmsg(ex.Message + Environment.NewLine); return; } } // ShowAlarm.Delay(100); //等待激光读取完成 bary1 = binHelper.toBin(string.Format("MS 0 0\r")); SendAndGetData(bary1, out buffer1, (uint)1 * 12, Laser.Down); str1 = new string(Encoding.Default.GetChars(buffer1)); double value1 = 0; if (str1.Contains("-----------")) //超出范围 { tbx_downLaser.Text = "NA"; } else { var listtmp1 = str1.Split(new string[] { "," }, StringSplitOptions.RemoveEmptyEntries).ToList().ConvertAll((s) => double.Parse(s)); //格式化成mm value1 = Convert.ToInt32(listtmp1[0]); value1 = value1 / 1000000; tbx_downLaser.Text = value1.ToString(); } //计算和 double sum = value +value1 ; tbx_addValue.Text = sum.ToString(); Thread.Sleep(10); } } public bool workInfoFromDisk() { try { var res = Serialize.FileDeSerialize(AppDomain.CurrentDomain.BaseDirectory + "autoCaliSetting"); if (null != res) { configdata = res as winData; return true; } return false; } catch (Exception ex) { return false; } } public bool workInfoToDisk() { try { Serialize.FileSerialize(AppDomain.CurrentDomain.BaseDirectory + "autoCaliSetting", configdata); return true; } catch (Exception ex) { throw new ArgumentException(ex.Message); } } private void btnRead_Click(object sender, EventArgs e) { try { configdata.dataSum = int.Parse(tbSum.Text); configdata.laserIP = tbLaserIP.Text; configdata.laserPort = int.Parse(tbLaserPort.Text); workInfoToDisk(); readLaserData(configdata.laserIP, configdata.dataSum); } catch (Exception ex) { MessageBox.Show(ex.Message); } } List<double> laserBufferUp = new List<double>(); List<double> laserBufferDown = new List<double>(); private void readLaserData(string ip, int readSum) { bool isConnect = false; Laser laser; if (ip == "192.168.250.51") { isConnect = isConnectUp; laserBufferUp.Clear(); laser = Laser.Up; } else { isConnect = isConnectDown; laserBufferDown.Clear(); laser = Laser.Down; } if (!isConnect) { try { InitPort(configdata.laserIP, configdata.laserPort, laser); outmsg("初始化成功!\r\n"); var bary = binHelper.toBin(string.Format("LS 1 {0}\r", readSum)); var buffer = new byte[] { }; SendAndGetData(bary, out buffer, (uint)bary.Length, laser); outmsg("读取数据 " + new string(Encoding.Default.GetChars(buffer))); } catch (Exception ex) { outmsg(ex.Message + Environment.NewLine); return; } } ShowAlarm.Delay(100); //等待激光读取完成 var bary1 = binHelper.toBin(string.Format("MS 0 0\r")); byte[] buffer1 = new byte[] { }; SendAndGetData(bary1, out buffer1, (uint)readSum * 12, laser); var str1 = new string(Encoding.Default.GetChars(buffer1)); List<double> listtmp = new List<double>(); try { listtmp = str1.Split(new string[] { "," }, StringSplitOptions.RemoveEmptyEntries).ToList().ConvertAll((s) => double.Parse(s)); } catch { } if (ip == "192.168.250.50") { laserBufferUp = listtmp; } else { laserBufferDown = listtmp; } outmsg(str1 + Environment.NewLine); } private void btnCalThickness_Click(object sender, EventArgs e) { var ao = new asynOperation(); ao.FuncListAdd(readLaserUpData); ao.FuncListAdd(readLaserDownData); var f1 = ao.AsynFunList(); outmsg(string.Format("厚度:{0}\r\n", calThickness())); } private double calThickness() { if (laserBufferUp.Count == laserBufferDown.Count) { var list1 = new List<double>(); for (int i = 0; i < laserBufferUp.Count; i++) { list1.Add(laserBufferUp[i] + laserBufferDown[i]); } return list1.Average(); } else { outmsg("激光上下头采集数据数量不相等!\r\n"); return 0; } } private bool readLaserUpData() { readLaserData("192.168.250.50", 10); return true; } private bool readLaserDownData() { readLaserData("192.168.250.51", 10); return true; } private void Form1_FormClosing(object sender, FormClosingEventArgs e) { try { configdata.dataSum = int.Parse(tbSum.Text); configdata.laserIP = tbLaserIP.Text; configdata.laserPort = int.Parse(tbLaserPort.Text); workInfoToDisk(); Close(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } Object obj = new Object(); bool SendAndGetData(Byte[] readCmd, out Byte[] rcvByte, uint byteLength, Laser laser) { lock (obj) { rcvByte = new byte[byteLength]; if (laser == Laser.Up) { if (!Clients[0].SendMsg(readCmd)) return false; if (!Clients[0].ReceiveMsg(out rcvByte, byteLength)) return false; } else { if (!Clients[1].SendMsg(readCmd)) return false; if (!Clients[1].ReceiveMsg(out rcvByte, byteLength)) return false; } return true; } } private void outmsg(string msg) { if (richTextBox1.InvokeRequired) { richTextBox1.Invoke(new Action(() => { richTextBox1.AppendText(msg); })); } else { richTextBox1.AppendText(msg); } } SocketClient[] Clients = new SocketClient[2]; bool isConnectUp = false; bool isConnectDown = false; public void InitPort(string IpAdrr, int Port, Laser laser) { bool f1 = true; try { do { if (laser == Laser.Up) { SocketClient SktClientUpLaser = new SocketClient(); if (!SktClientUpLaser.Connecting(IpAdrr, Port)) { outmsg(string.Format("连接激光 IP {0}, 端口 {1} 时失败!", IpAdrr, Port)); f1 = false; break; } if (!SktClientUpLaser.SetReceiveTimeOut(4000)) { f1 = false; break; } Clients[0] = SktClientUpLaser; } else { SocketClient SktClientDownLaser = new SocketClient(); if (!SktClientDownLaser.Connecting(IpAdrr, Port)) { outmsg(string.Format("连接激光 IP {0}, 端口 {1} 时失败!", IpAdrr, Port)); f1 = false; break; } if (!SktClientDownLaser.SetReceiveTimeOut(4000)) { f1 = false; break; } Clients[1] = SktClientDownLaser; // var bary = binHelper.toBin(string.Format("LS 1 {0}\r", 1)); //var buffer = new byte[] { }; //SendAndGetData(bary, out buffer, (uint)bary.Length, Laser.Down); //outmsg("读取数据 " + new string(Encoding.Default.GetChars(buffer1))); } } while (false); if (IpAdrr.Equals("192.168.250.51")) isConnectUp = f1; else isConnectDown = f1; } catch (Exception ex) { outmsg(ex.Message); if (IpAdrr.Equals("192.168.250.50")) isConnectUp = false; else isConnectDown = false; } } public void Close() { try { if (Clients[0] != null) { if (Clients[0].SockClient != null && Clients[0].SockClient.Connected) Clients[0].SockClient.Close(); Clients[0].Dispose(); } if (Clients[1] != null) { if (Clients[1].SockClient != null && Clients[1].SockClient.Connected) Clients[1].SockClient.Close(); Clients[1].Dispose(); } } catch (Exception ex) { MessageBox.Show(ex.Message); } } } [Serializable] public class winData { public int dataSum { get; set; } public string laserIP { get; set; } public int laserPort { get; set; } } public enum Laser { Up, Down, } }
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: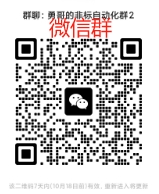
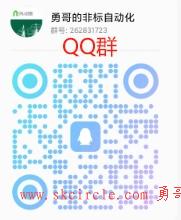
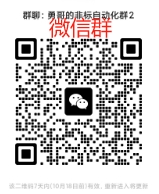
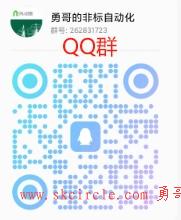