已知直线的起始端点与终止端点,移动到指定点的位置。
这个必须要考虑直线有可能是任意角度的问题。
#代码如下:
要注意,下面的代码需要安装netMarketing类库。
using HalconDotNet; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsApplication1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { double x1=0,y1=0,x2=0,y2=0; MoveLine(3182, 2449, 3114.10, 1860.05, 2962.10, 3420.20, out x1, out y1, out x2, out y2); richTextBox1.Text = string.Format("{0},{1},{2},{3}",x1,y1,x2,y2); } /// <summary> /// 移动线段到指定点 /// </summary> /// <param name="lineStartPointRow">点行</param> /// <param name="lineStartPointCol">点列</param> /// <param name="lineStartPointRow">待移动线段起点行</param> /// <param name="lineStartPointCol">待移动线段起点列</param> /// <param name="lineEndPointRow">待移动线段终点行</param> /// <param name="lineEndPointCol">待移动线段终点列</param> /// <param name="resultLineStartPointRow">移动后线段起点行</param> /// <param name="resultLineStartPointCol">移动后线段起点列</param> /// <param name="resultLineEndPointRow">移动后线段终点行</param> /// <param name="resultLineEndPointCol">移动后线段终点列</param> private void MoveLine(double pointRow, double pointCol, double lineStartPointRow, double lineStartPointCol, double lineEndPointRow, double lineEndPointCol, out double resultLineStartPointRow, out double resultLineStartPointCol, out double resultLineEndPointRow, out double resultLineEndPointCol) { resultLineStartPointRow = 0; resultLineStartPointCol = 0; resultLineEndPointRow = 0; resultLineEndPointCol = 0; //首先得到垂点 HTuple pitchRow, pitchCol; HOperatorSet.ProjectionPl(pointRow, pointCol, lineStartPointRow, lineStartPointCol, lineEndPointRow, lineEndPointCol, out pitchRow, out pitchCol); //得到垂点距离起点A的距离 HTuple distanceA; HOperatorSet.DistancePp(pitchRow, pitchCol, lineStartPointRow, lineStartPointCol, out distanceA); //得到垂点距离终点B的距离 HTuple distanceB; HOperatorSet.DistancePp(pitchRow, pitchCol, lineEndPointRow, lineEndPointCol, out distanceB); //根据移动位置点,距离A,和线段角度求结果线段的起点 double angle; HTuple temp; HOperatorSet.AngleLx(pitchRow, pitchCol, lineStartPointRow, lineStartPointCol, out temp); angle = (double)temp; double drow = 0; double dcolumn = 0; if (0 <= angle * (180 / Math.PI) && angle * (180 / Math.PI) <= 90) { dcolumn = Math.Abs(((double)distanceA) * Math.Cos(angle)); drow = Math.Abs(((double)distanceA) * Math.Sin(angle)); resultLineStartPointRow = pointRow - drow; resultLineStartPointCol = pointCol + dcolumn; } else if (angle * (180 / Math.PI) > 90 && angle * (180 / Math.PI) <= 180) { dcolumn = ((double)distanceA) * Math.Cos((Math.PI - angle)); drow = ((double)distanceA) * Math.Sin((Math.PI - angle)); resultLineStartPointRow = pointRow - drow; resultLineStartPointCol = pointCol - dcolumn; } else if (angle * (180 / Math.PI) < 0 && angle * (180 / Math.PI) >= -90) { dcolumn = ((double)distanceA) * Math.Cos(Math.Abs(angle)); drow = ((double)distanceA) * Math.Sin(Math.Abs(angle)); resultLineStartPointRow = pointRow + drow; resultLineStartPointCol = pointCol + dcolumn; } else if (angle * (180 / Math.PI) < -90 && angle * (180 / Math.PI) >= -180) { dcolumn = Math.Abs(((double)distanceA) * Math.Cos(angle + Math.PI)); drow = Math.Abs(((double)distanceA) * Math.Sin(angle + Math.PI)); resultLineStartPointRow = pointRow + drow; resultLineStartPointCol = pointCol - dcolumn; } //根据移动位置点,距离B,和线段角度求结果线段的终点 HOperatorSet.AngleLx(pitchRow, pitchCol, lineEndPointRow, lineEndPointCol, out temp); angle = (double)temp; if (0 <= angle * (180 / Math.PI) && angle * (180 / Math.PI) <= 90) { dcolumn = Math.Abs(((double)distanceB) * Math.Cos(angle)); drow = Math.Abs(((double)distanceB) * Math.Sin(angle)); resultLineEndPointRow = pointRow - drow; resultLineEndPointCol = pointCol + dcolumn; } else if (angle * (180 / Math.PI) > 90 && angle * (180 / Math.PI) <= 180) { dcolumn = ((double)distanceB) * Math.Cos((Math.PI - angle)); drow = ((double)distanceB) * Math.Sin((Math.PI - angle)); resultLineEndPointRow = pointRow - drow; resultLineEndPointCol = pointCol - dcolumn; } else if (angle * (180 / Math.PI) < 0 && angle * (180 / Math.PI) >= -90) { dcolumn = ((double)distanceB) * Math.Cos(Math.Abs(angle)); drow = ((double)distanceB) * Math.Sin(Math.Abs(angle)); resultLineEndPointRow = pointRow + drow; resultLineEndPointCol = pointCol + dcolumn; } else if (angle * (180 / Math.PI) < -90 && angle * (180 / Math.PI) >= -180) { dcolumn = (Math.Abs((double)distanceB) * Math.Cos((angle + Math.PI))); drow = (Math.Abs((double)distanceB) * Math.Sin((angle + Math.PI))); resultLineEndPointRow = pointRow + drow; resultLineEndPointCol = pointCol - dcolumn; } } } }
为了验证这个结果,编写halcon代码如下:
read_image (Image, 'C:/Users/Administrator/Desktop/尺寸机/建模/395876G-3-HWR - 副本2.bmp') dev_open_window_fit_image(Image, 0, 0, -1, -1, WindowHandle) dev_display(Image) dev_set_color('green') disp_line(WindowHandle, 3114.10, 1860.05, 2962.10, 3420.20) disp_line(WindowHandle, 3238.201393,1872.1407686,3086.20139298163,3432.29076802436)
结果如下图所示:
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: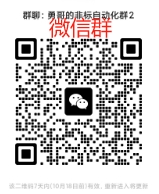
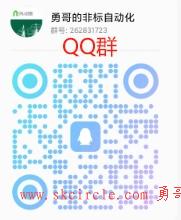
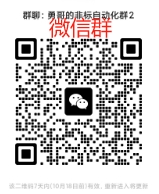
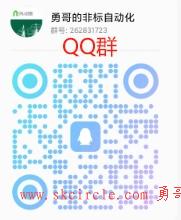