这篇勇哥要熟悉一下QT的信号槽怎么样快速实现。
想要快速实现就不能完全敲代码,可以借助于QT的UI编辑器来完成。
另外通过常见的QCommboBox控件来熟悉控件的操作。还有多个窗口是如何调用。
代码:
QtForOpencv.h
#pragma once #pragma execution_character_set("utf-8") #include <QtWidgets/QWidget> #include "ui_QtForOpencv.h" #include <QLabel> #include "QtOpenTxt.h" class QtForOpencv : public QWidget { Q_OBJECT public: QtForOpencv(QWidget *parent = Q_NULLPTR); private: Ui::QtForOpencvClass ui; QtOpenTxt * openfile; private slots: void slotInitItems(); //初始化下拉列表框 void slotClearItems(); //清除列表框所有项 void slotInsertItem(); //插入新项 void slotDeleteItem(); //插队某项 void slotDisplayItems(); //在文本框中显示所有信息,包含附加数据 void slotCurrentIndexChanged(int val); void slotCurrentIndexChanged(const QString &val); void slotOpenText(); void slotOpentxtNewWin(); public: QLabel* imgLabel; };
QtForOpencv.cpp
#include "QtForOpencv.h" #include <opencv2\opencv.hpp> #include <opencv2\core.hpp> #include <opencv2\imgcodecs.hpp> #include <opencv2\imgproc.hpp> #include <qdebug.h> #include <qfiledialog.h> #include <qmessagebox.h> using namespace cv; using namespace std; QtForOpencv::QtForOpencv(QWidget *parent) : QWidget(parent) { ui.setupUi(this); //这个个信号槽勇哥改成在界面上去操作了。所以这里没有必须要再写了。 //connect(ui.btnInit, SIGNAL(clicked()), this, SLOT(slotInitItems())); //其它的信号槽都用代码写出来。 connect(ui.btnClearItem, SIGNAL(clicked()), this, SLOT(slotClearItems())); connect(ui.btnInsert, SIGNAL(clicked()), this, SLOT(slotInsertItem())); connect(ui.btnDel, SIGNAL(clicked()), this, SLOT(slotDeleteItem())); connect(ui.btnDisplayInfo, SIGNAL(clicked()), this, SLOT(slotDisplayItems())); connect(ui.cbxList, SIGNAL(currentIndexChanged(int)), SLOT(slotCurrentIndexChanged(int))); connect(ui.cbxList, SIGNAL(currentIndexChanged(const Qstring &)), SLOT(slotCurrentIndexChanged(const QString &))); waitKey(0); } void QtForOpencv::slotInitItems() { QMap<QString, int> map; map.insert("张三", 81); map.insert("李四", 88); map.insert("王五", 73); map.insert("赵六", 91); map.insert("李七", 90); qDebug() << "slotInitItems"; QIcon icon(":/QtForOpencv/E:/程序开发用图标/程序开发用图标/编程1000多个图标/Icon/007A.ICO"); ui.cbxList->clear(); foreach(const QString name,map.keys()) { if (ui.chkIsIcon->isChecked()) ui.cbxList->addItem(icon, name, map.value(name)); else ui.cbxList->addItem(name, map.value(name)); } } void QtForOpencv::slotClearItems() //清除列表框所有项 { ui.cbxList->clear(); } void QtForOpencv::slotInsertItem() //插入新项 { int pos = ui.spinBoxInserPos->value(); QIcon icon(":/QtForOpencv/E:/程序开发用图标/程序开发用图标/编程1000多个图标/Icon/014A.ICO"); if (ui.chkIsIcon->isChecked()) ui.cbxList->insertItem(pos, icon, "new item", 88); else ui.cbxList->insertItem(pos, "new item", 88); } void QtForOpencv::slotDeleteItem() //插队某项 { int pos = ui.spinBoxDeletePos->value(); ui.cbxList->removeItem(pos); } void QtForOpencv::slotDisplayItems() //在文本框中显示所有信息,包含附加数据 { for (int i = 0; i < ui.cbxList->count(); i++){ ui.txtMsg->appendPlainText( QString("index:%1 | text:%2 | score:%3") .arg(i) .arg(ui.cbxList->itemText(i)) .arg(ui.cbxList->itemData(i).toString()) ); } ui.txtMsg->appendPlainText("============================"); } void QtForOpencv::slotCurrentIndexChanged(int val){ ui.txtMsg->appendPlainText(QString("current index changed: index=%1").arg(val)); } void QtForOpencv::slotCurrentIndexChanged(const QString &val){ ui.txtMsg->appendPlainText(QString("current index changed: text=%1").arg(val)); } void QtForOpencv::slotOpentxtNewWin() { openfile = new QtOpenTxt; openfile->show();//openfile窗口显示 //this->hide();//本窗口隐藏 } void QtForOpencv::slotOpenText() { qDebug() << "slotOpenText"; QString filename; //限定只能打开*.txt文件 filename = QFileDialog::getOpenFileName(this, tr("Open File"), "", tr("*.txt")); if (!filename.isNull()) { //filename是选择的文件名 QFile file(filename); //如果读文件错误,要输出提示信息 if (!file.open(QFile::ReadOnly | QFile::Text)) { QMessageBox::warning(this, tr("Error"), tr("read file error:&1").arg(file.errorString())); return; } //文件流 QTextStream in(&file); //QApplication::setOverrideCursor(Qt::WaitCursor); //将读入的文件信息显示到textEdit中 ui.txtMsg->appendPlainText(in.readAll()); file.close(); } else { qDebug() << "取消"; } }
QtOpenTxt.h
#pragma once #include <QWidget> #include "ui_QtOpenTxt.h" class QtOpenTxt : public QWidget { Q_OBJECT private slots: void slotOpentxt(); void slotWinQuit(); public: QtOpenTxt(QWidget *parent = Q_NULLPTR); ~QtOpenTxt(); private: Ui::QtOpenTxt ui; };
QtOpenTxt.cpp
#include "QtOpenTxt.h" #include <qtextstream.h> #include <qfiledialog.h> #include <qfile.h> #include <qmessagebox.h> #include "QtForOpencv.h" #include <qdebug.h> QtOpenTxt::QtOpenTxt(QWidget *parent) : QWidget(parent) { ui.setupUi(this); } QtOpenTxt::~QtOpenTxt() { } void QtOpenTxt::slotOpentxt(){ QString filename; //限定只能打开*.txt文件 filename = QFileDialog::getOpenFileName(this, tr("Open File"), "", tr("*.txt")); if (!filename.isNull()) { //filename是选择的文件名 QFile file(filename); //如果读文件错误,要输出提示信息 if (!file.open(QFile::ReadOnly | QFile::Text)) { QMessageBox::warning(this, tr("Error"), tr("read file error:&1").arg(file.errorString())); return; } //文件流 QTextStream in(&file); //QApplication::setOverrideCursor(Qt::WaitCursor); //将读入的文件信息显示到textEdit中 ui.txtMsg->setText(in.readAll()); file.close(); } else { qDebug() << "取消"; } } void QtOpenTxt :: slotWinQuit(){ /* *同样这里暂时先给出一段弹出提示返回成功的弹窗代码 *稍后on_backbutton_clicked()函数需要移动到别处,此处就不需要了 */ QMessageBox message(QMessageBox::NoIcon, "Tip", "Back successfully "); message.exec(); //QtForOpencv *program = new QtForOpencv; //program->show();//program窗口显示 //this->hide();//本窗口隐藏 //this->close(); this->close(); }
几点说明:
(1)UI设计器上做信号槽的方法,就是用红框处的工具。
(2)在界面上访问控件的方法
首选你在UI设计器中自己把控件命名。
然后在代码里,就是以ui. 控件的名字,来访问控件了。
然而,你是不是即使这样做了, ui.btnClearItem 仍然不存呢?
这是因为你UI编辑器完成后,你得在vs中把这个UI编译一下。
这时候你会发现vs提示文件被更新了,出现这个就对了,因为Ui那边的更新被发现了。
然后就发现ui.btnClearItem这个可以出现在语法补全的列表中了。
如果你发现即重新编译ui,也不行,试试下面这个按钮,显示全部文件,然后再编译ui, 应该就可以了。
当然,如果还是不行,终极的办法就是重新打开工程,再编译肯定就可以了。
(3) QString类的arg方法
详细见另一篇贴子:
其它的就没有啥子好说了。总得来讲,QT这么做确实让UI控件易于使用了。有了几分C#做界面的感觉了。
接下来就要熟悉一下工业设备软件中常用的一些控件,在QT中对应的控件了。
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
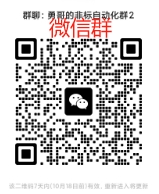
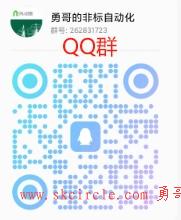