经过两个多小时的google和试探,终于搞定了一段代码.通过这个代码可以控制当前线程的CPU使用量.下面是代码:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using FILETIME = System.Runtime.InteropServices.ComTypes.FILETIME; namespace WindowsFormsApplication1 { public class CPU { private int mUsage = 20; public int MaximumUsage { get { return this.mUsage; } set { if (value > 0 && value < 100) { this.mUsage = value; } } } private long LastTotalThreadTime { get; set; } private long LastTotalSystemTime { get; set; } public CPU(int usage) { this.MaximumUsage = usage; this.LastTotalThreadTime = 0; this.LastTotalSystemTime = 0; this.LastTotalSystemTime = this.GetSystemTime(); this.LastTotalThreadTime = this.GetThreadTime(); } public void Sleep() { long systemTime, threadTime; systemTime = this.GetSystemTime(); threadTime = this.GetThreadTime(); if (threadTime > 0) { long sleepTimes = ((threadTime - this.LastTotalThreadTime) * this.MaximumUsage) - (systemTime - this.LastTotalSystemTime); sleepTimes /= 10000; if (sleepTimes > 0) { TimeSpan ts = TimeSpan.FromMilliseconds(sleepTimes); Thread.Sleep(ts); this.LastTotalThreadTime = threadTime; this.LastTotalSystemTime = systemTime; } } } [DllImport("kernel32.dll", SetLastError = true)] private static extern bool GetSystemTimes( out FILETIME lpIdleTime, out FILETIME lpKernelTime, out FILETIME lpUserTime ); [DllImport("kernel32.dll", SetLastError = true)] private static extern bool GetThreadTimes(IntPtr hThread, out FILETIME lpCreationTime, out FILETIME lpExitTime, out FILETIME lpKernelTime, out FILETIME lpUserTime); [DllImport("kernel32.dll")] private static extern IntPtr GetCurrentThread(); private long GetLong(FILETIME ft) { long result = ((long)ft.dwHighDateTime << 32) + ft.dwLowDateTime; return result; } private long GetSystemTime() { long result = this.LastTotalSystemTime; FILETIME ftIdleTime, ftKernelTime, ftUserTime; if (GetSystemTimes(out ftIdleTime, out ftKernelTime, out ftUserTime)) { long idleTime = this.GetLong(ftIdleTime); long kernelTime = this.GetLong(ftKernelTime); long userTime = this.GetLong(ftUserTime); result = idleTime + kernelTime + userTime; } return result; } private long GetThreadTime() { long result = this.LastTotalThreadTime; FILETIME thCreationTime, thExitTime, thKernelTime, thUserTime; IntPtr threadId = GetCurrentThread(); if (GetThreadTimes(threadId, out thCreationTime, out thExitTime, out thKernelTime, out thUserTime)) { long kernelTime = this.GetLong(thKernelTime); long userTime = this.GetLong(thUserTime); result = kernelTime + userTime; } return result; } } }
没有太多的技术含量,就是调用了3个Windows的API然后计算一下当前线程占用的时间和整个机器运行的时间之间的比值,比值超过某个特定的域就让当前线程休息一会.休眠的时间也是根据那个CPU占用率计算出来的,这样可以将当前线程的CPU占有率大约控制在一个指定的值周围。
下面是如何使用的代码:
CPU cpu = new CPU(20); while (true) { long result = 0; int times = new Random().Next(10000); for (int i = 0; i < times; i++) { result += i; } cpu.Sleep(); }
————————————————
版权声明:本文为CSDN博主「zhuliangxiong」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。
原文链接:https://blog.csdn.net/zhuliangxiong/article/details/4097889
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: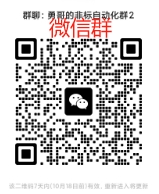
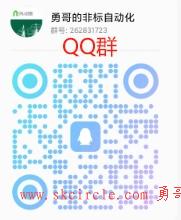
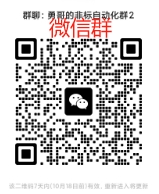
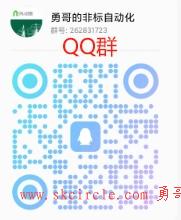